SDK Payment process
Web View for 3DS
The diagram below shows the SDK Payment payment process with 3DS redirect via Web View.
- Client creates an order.
- Mobile Server registers that order in Payment Gateway via register.do. Use
returnUrl
parameter as a marker to close Web View after redirect from ACS. - Mobile Server receives a unique order number
mdOrder
in response. - Mobile App initiates SDK Payment to collect Client's payment data.
- Client fills in payment data.
- SDK sends encrypted payment data (seToken) to Payment Gateway.
- Payment Gateway makes a payment.
- If it's required, Payment Gateway handles 3DS Secure communication.
- To process 3DS 2 payments, we recommend you to use web view redirect (
use3ds2sdk=false
).
- Payment Gateway sends callback notification to Merchant server if it's configured for the merchant.
- Mobile Server checks the final payment status via getOrderStatusExtended.do.
- Mobile App shows payment result to the client.
iOS
The project setup is described below. Framework can be installed manually.
SDKPayment is based on ThreeDSSDK, SDKForms frameworks. Therefore they are required for import.
To install frameworks manually, download and add them to the project.
Instructions for setting up each module can be found below:
iOS Integration
SDKForms.framework integration
You can integrate SDKForms.framework in the following way:
- Add
SDKForms.framework
andSDKCore.framework
manually.
SDKForms.framework
Download the latest version of the frameworks here.
Take the
SDKCore.framework
andSDKForms.framework
files and add them to the project folder.
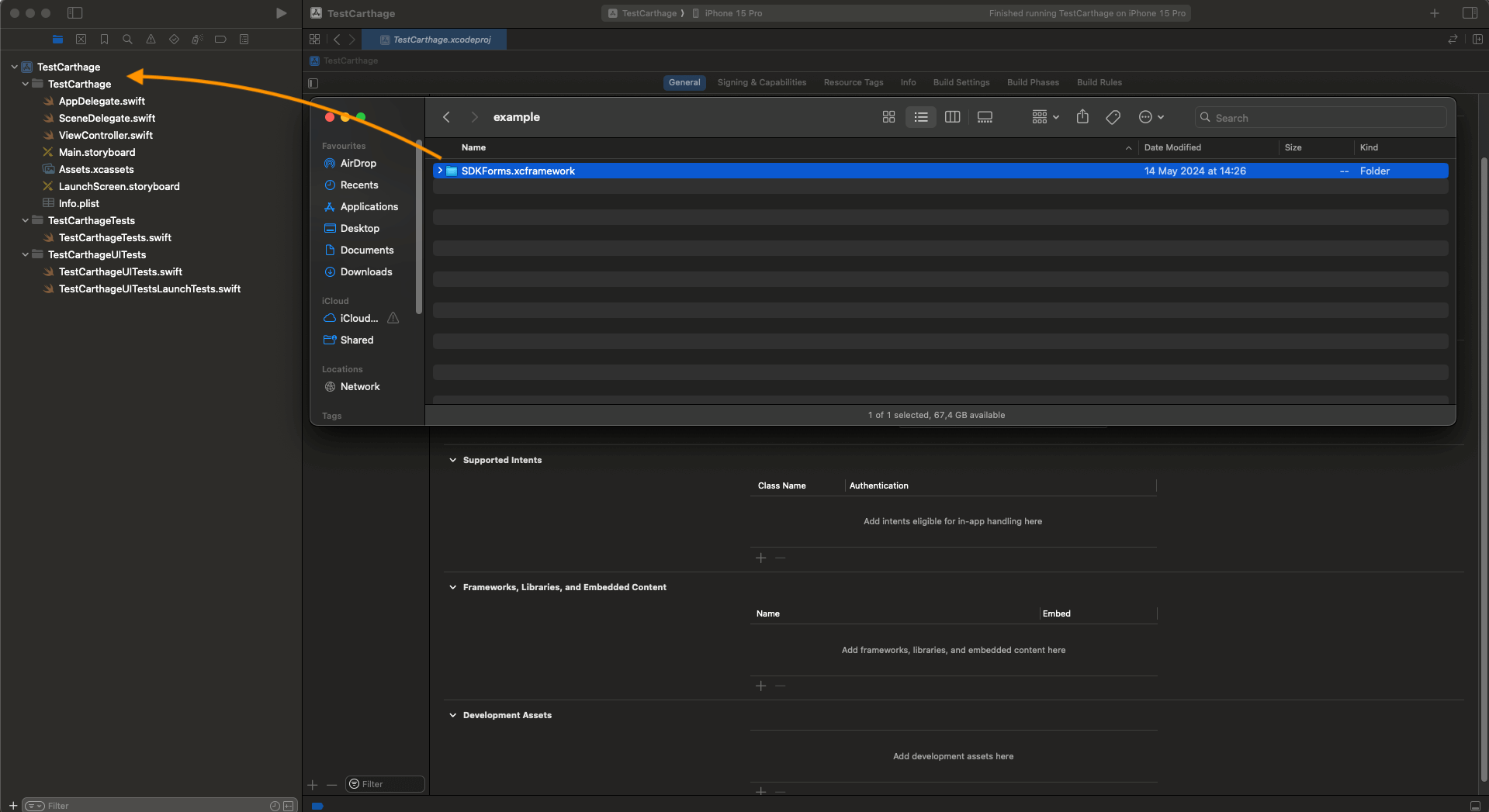
- Open Targets -> General -> Frameworks, Libraries, and Embedded Content. For
SDKCore.framework
andSDKForms.framework
, in the Embed column, changeDo not Embed
toEmbed & Sign
.
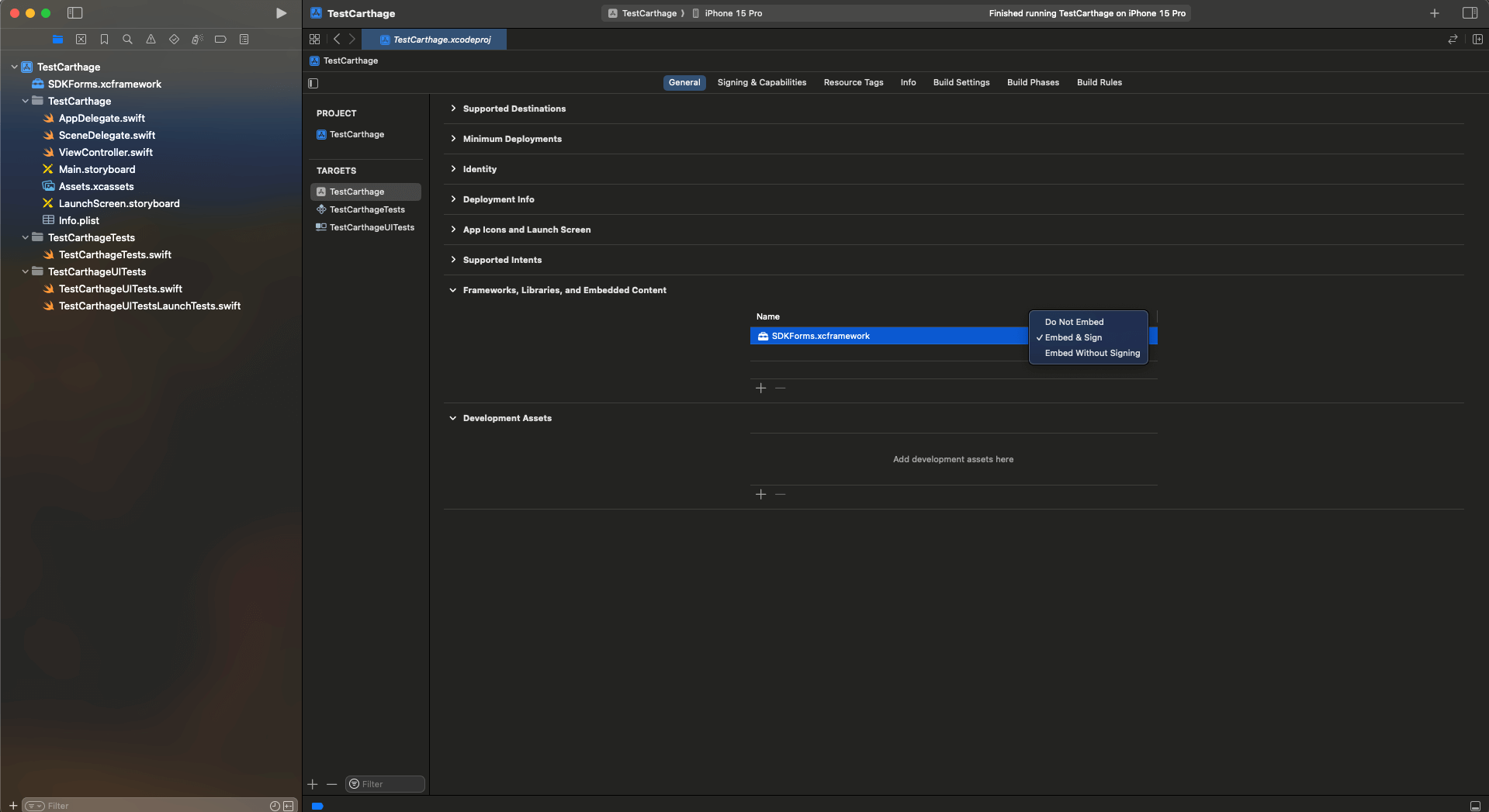
Once done, import the framework in the ViewController.swift
file.
SDKPayment.framework integration
SDKPayment.framework
- Download the latest version of the frameworks here.
- Take the downloaded files and add them to the project folder.
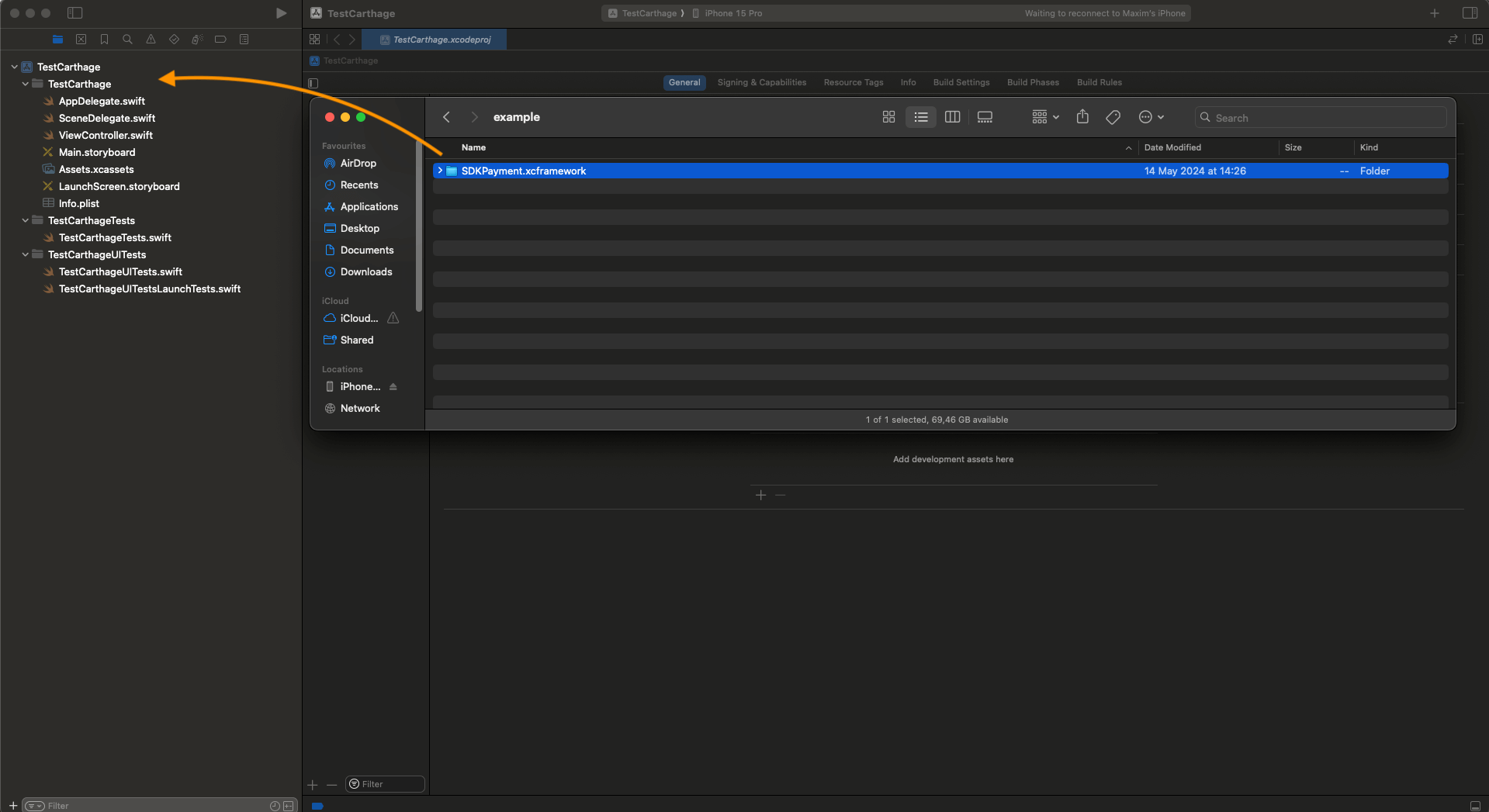
- Open Targets -> General -> Frameworks, Libraries, and Embedded Content. For the frameworks, in the Embed column, change
Do not Embed
toEmbed & Sign
.
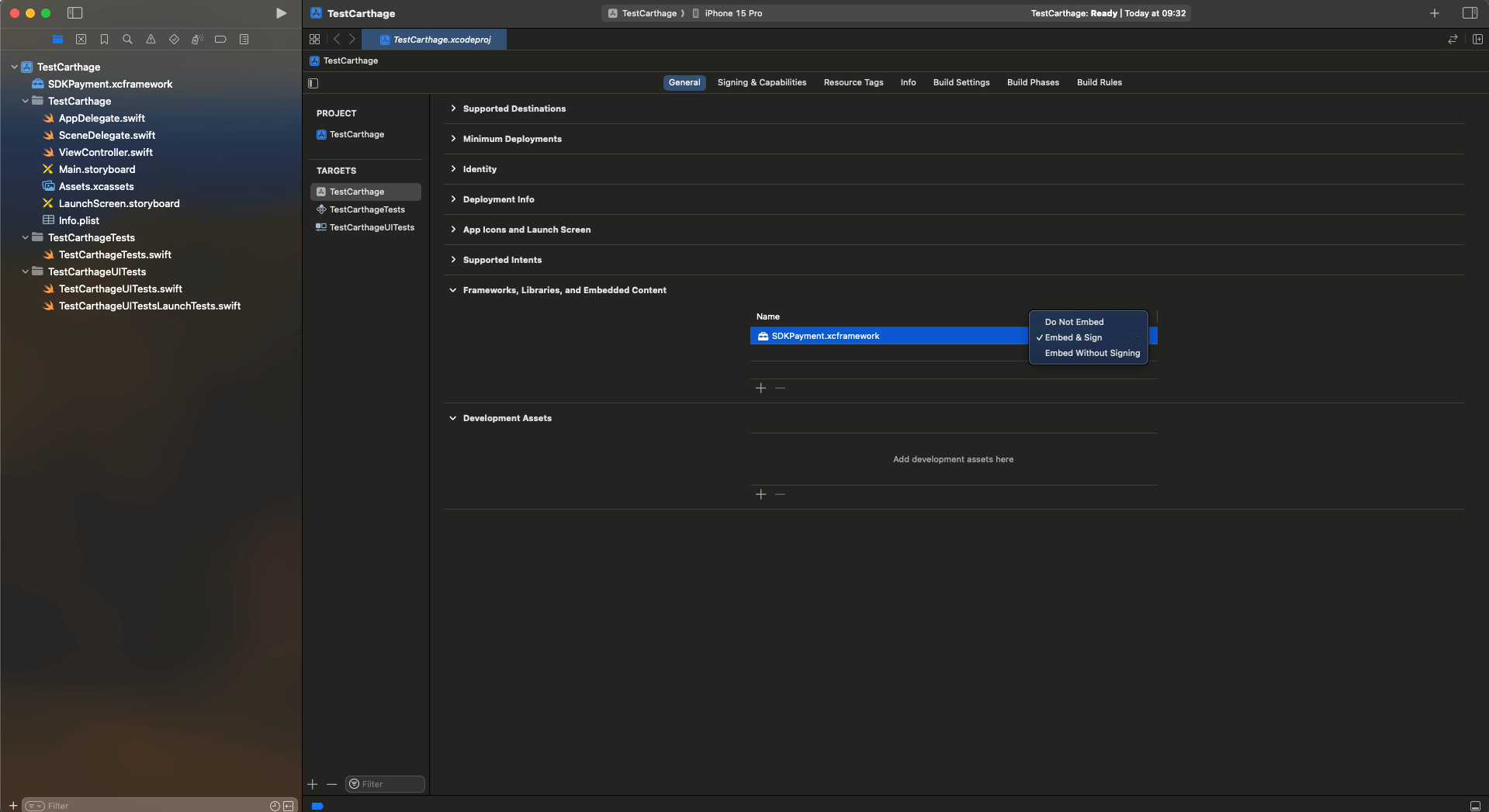
Once done, import framework in the ViewController.swift
file.
//ViewController.swift
...
import SDKPayment
...
How to work with API v2
SDK Configuration
For initialization, it is necessary to set the payment gateway server address.
final class MainViewController: UIViewController {
private func checkout() {
SdkPayment.initialize(
sdkPaymentConfig: SDKPaymentConfig(
baseURL: "\(baseApiUrl)",
use3DSConfig: .noUse3ds2sdk,
keyProviderUrl: "\(baseApiUrl)/se/keys.do"
)
)
...
}
}
When using API v2, you need to register a new session
checkout.
When registering a session
, the request must contain successUrl
and failureUrl
parameters equal to "sdk://done".
To use the checkoutWithBottomSheet()
method, you need to create CheckoutConfig
with sessionid
.
final class MainViewController: UIViewController {
private func checkout() {
...
// Creating `CheckoutConfig`
let checkoutConfig = CheckoutConfig(id: .sessionId(id: sessionId))
SdkPayment.shared.checkoutWithBottomSheet(
controller: navigationController!,
checkoutConfig: checkoutConfig,
callbackHandler: self
)
}
}
Payment result processing
For MainViewController
, you need to implement the requirements of ResultPaymentCallback
.
extension MainViewController: ResultPaymentCallback {
typealias T = PaymentResult
func onResult(result: PaymentResult) {
print("\(result.isSuccess) \(result.paymentId)")
}
}
On successful payment, a PaymentResult
object is returned containing the isSuccess
boolean field with
the result of the payment and optional exception
field.
Screen samples
Paying with a new card
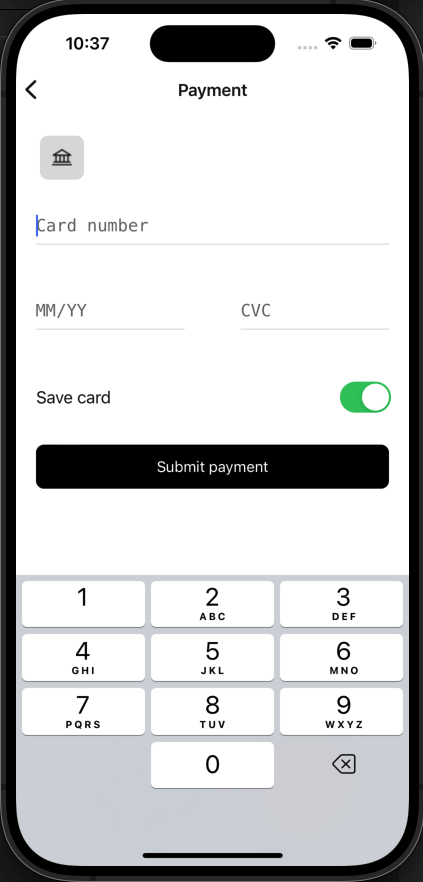
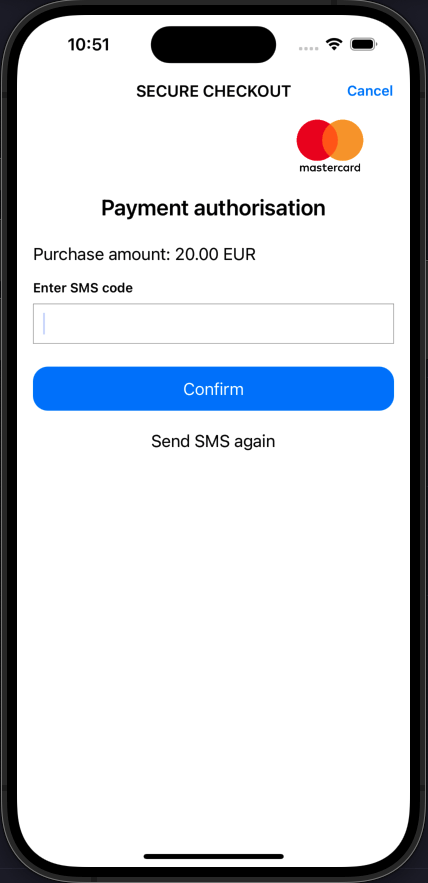
Paying with a bound card
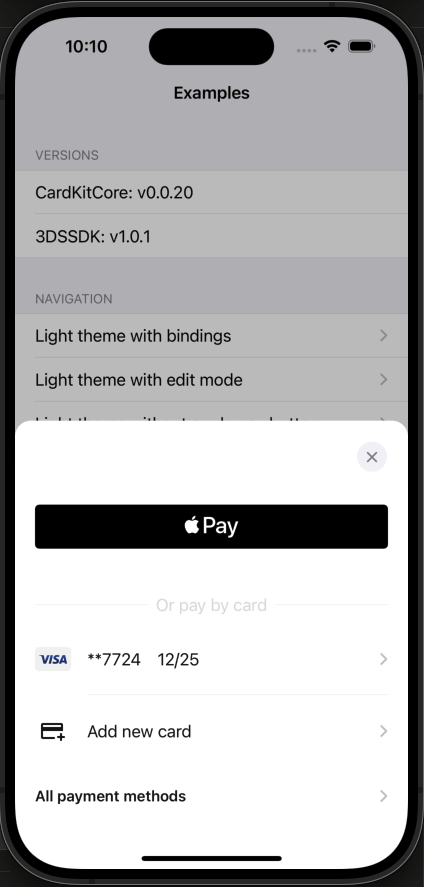
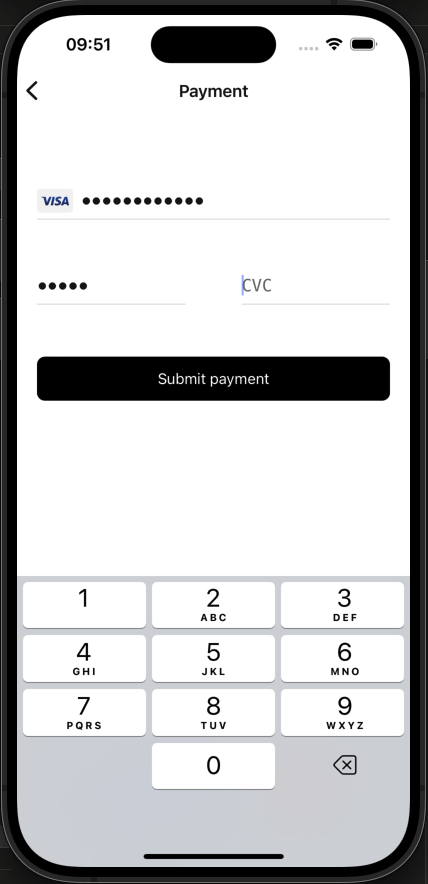
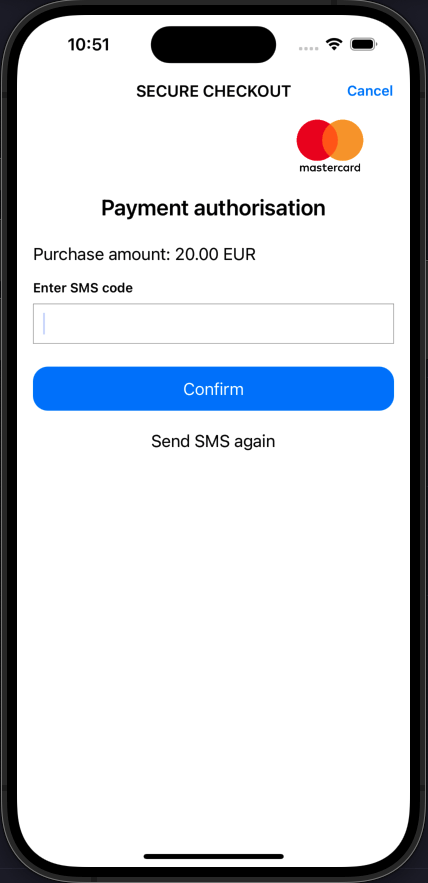
Logging
Internal processes are logged with the SDKPayment
tag.
You can also log your processes.
Logging is available through the Logger.shared
object.
- To add log- interfaces you should call the
Logger
-methodaddLogInterface()
.
Example for logging:
...
final class LoggerImplementation: LogInterface {
func log(class: AnyClass, tag: String, message: String, exception: (any Error)?) {
// Do something for logging
}
}
Logger.shared.addLogInterface(logger: LoggerImplementation())
...
The default tag is SDKPayment
. You can set your own one if you like.
- To log your own events, you should call the
Logger.shared
-methodlog()
.
Example:
...
Logger.shared.log(classMethod: type(of: self),
tag: "MyTag",
message: "My process...",
exception: nil)
...
How to work with API V1
SDK configuration
For initialization, it is necessary to set the payment gateway server address.
final class MainViewController: UIViewController {
private func checkout() {
SdkPayment.initialize(
sdkPaymentConfig: SDKPaymentConfig(
baseURL: "\(baseApiUrl)",
use3DSConfig: .noUse3ds2sdk,
keyProviderUrl: "\(baseApiUrl)/se/keys.do"
)
)
...
}
}
When using API v1, you need to register new order
.
When registering an order
, the request must contain the returnUrl
parameter equal to "sdk://done".
To use the checkoutWithBottomSheet()
method, you need to create CheckoutConfig
with mdOrder
.
final class MainViewController: UIViewController {
private func checkout() {
...
// Creating `CheckoutConfig`
let checkoutConfig = CheckoutConfig(id: .mdOrder(id: mdOrder))
SdkPayment.shared.checkoutWithBottomSheet(
controller: navigationController!,
checkoutConfig: checkoutConfig,
callbackHandler: self
)
}
}
Payment result processing
For MainViewController
, you need to implement the requirements of ResultPaymentCallback
.
extension MainViewController: ResultPaymentCallback {
typealias T = PaymentResult
func onResult(result: PaymentResult) {
print("\(result.isSuccess) \(result.paymentId)")
}
}
Android
SDKPayment is based on ThreeDSSDK, SDKForms frameworks. Therefore, they are required for import.
Android Integration
Connecting to a Gradle project by adding .aar library files
You must add the sdk_forms-release.aar
library file to the libs
folder, then specify the
dependency of the added library.
build.gradle.kts
allprojects {
repositories {
// ...
flatDir {
dirs("libs")
}
}
}
dependencies {
// dependency is mandatory to add
implementation(group = "", name = "sdk_forms-release", ext = "aar")
implementation("androidx.cardview:cardview:1.0.0")
implementation("com.github.devnied.emvnfccard:library:3.0.1")
implementation("com.caverock:androidsvg-aar:1.4")
implementation("io.card:android-sdk:5.5.1")
implementation("com.google.android.gms:play-services-wallet:18.0.0")
}
build.gradle
allprojects {
repositories {
// ...
flatDir {
dirs 'libs'
}
}
}
dependencies {
// dependency is mandatory to add
implementation(group = "", name = "sdk_forms-release", ext = "aar")
implementation("androidx.cardview:cardview:1.0.0")
implementation("com.github.devnied.emvnfccard:library:3.0.1")
implementation("com.caverock:androidsvg-aar:1.4")
implementation("io.card:android-sdk:5.5.1")
implementation("com.google.android.gms:play-services-wallet:18.0.0")
}
Connecting to a Gradle project by adding .aar library files
You must add the sdk_payment-release.aar
library file to the libs
folder, then specify the
dependency of the added library.
build.gradle.kts
allprojects {
repositories {
// ...
flatDir {
dirs("libs")
}
}
}
dependencies {
// dependency is mandatory to add
implementation(group = "", name = "sdk_payment-release", ext = "aar")
}
build.gradle
allprojects {
repositories {
// ...
flatDir {
dirs 'libs'
}
}
}
dependencies {
// dependency is mandatory to add
implementation(group = "", name = "sdk_payment-release", ext = "aar")
}
How to work with API V2
SDK configuration
For initialization, it is necessary to set the payment gateway server address.
SDKPayment.init(
SDKPaymentConfig(
baseURL = "https://dev.bpcbt.com/payment/rest",
)
)
When using API v2, you need to register a new session
checkout.
When registering a sessionId
, the request must contain successUrl
and failureUrl
parameters equal to "sdk://done".
// A link to an activity or a fragment is required. Checkout config is required.
val checkoutConfig = CheckoutConfig.sessionId("sessionId")
SDKPayment.checkout(activity = this, checkoutConfig = checkoutConfig)
The base URL for accessing payment gateway methods and the root certificate for verification signatures
are specified through the object of the SDKPaymentConfig
class.
The checkout method is available in two variations, to be called from Activity
and Fragment
:
checkout(activity: Activity, checkoutConfig: CheckoutConfig)
checkout(fragment: Fragment, checkoutConfig: CheckoutConfig)
Payment result processing
For Activity
and Fragment
, you need to override the onActivityResult
method.
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
// Processing the result of the payment cycle.
SDKPayment.handleCheckoutResult(requestCode, data, object :
ResultPaymentCallback<PaymentData> {
override fun onResult(result: PaymentResult) {
// check payment result
}
})
}
On successful payment, a PaymentResult
object is returned containing the isSuccess
boolean field with the result of the payment and optional exception
field.
How to work with API V1
SDK configuration
For initialization, it is necessary to set the payment gateway server address.
SDKPayment.init(
SDKPaymentConfig(
baseURL = "https://dev.bpcbt.com/payment/rest",
)
)
When using API v1, you need to register a new order
. When registering an order
, the request must contain returnUrl
and no other parameters with Url, like failUrl
. returnUrl
must be equal to "sdk://done".
// A link to an activity or a fragment is required. Checkout config is required.
val checkoutConfig = CheckoutConfig.MdOrder("eecbbe96-973e-422e-a220-e9fa8d6cb124")
SDKPayment.checkout(activity = this, checkoutConfig = checkoutConfig)
The base URL for accessing payment gateway methods and the root certificate for verification signatures
are specified through the object of the SDKPaymentConfig
class.
The checkout method is available in two variations, to be called from Activity
andFragment
:
checkout(activity: Activity, checkoutConfig: CheckoutConfig)
checkout(fragment: Fragment, checkoutConfig: CheckoutConfig)
Payment result processing
For Activity
andFragment
, you need to override the onActivityResult
method.
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
// Processing the result of the payment cycle.
SDKPayment.handleCheckoutResult(requestCode, data, object :
ResultPaymentCallback<PaymentData> {
override fun onResult(result: PaymentResult) {
// check payment result
}
})
}
On successful payment, a PaymentResult
object is returned containing the isSuccess
boolean field with the result of the payment and optional exception
field.
Screen samples
Paying with a new card
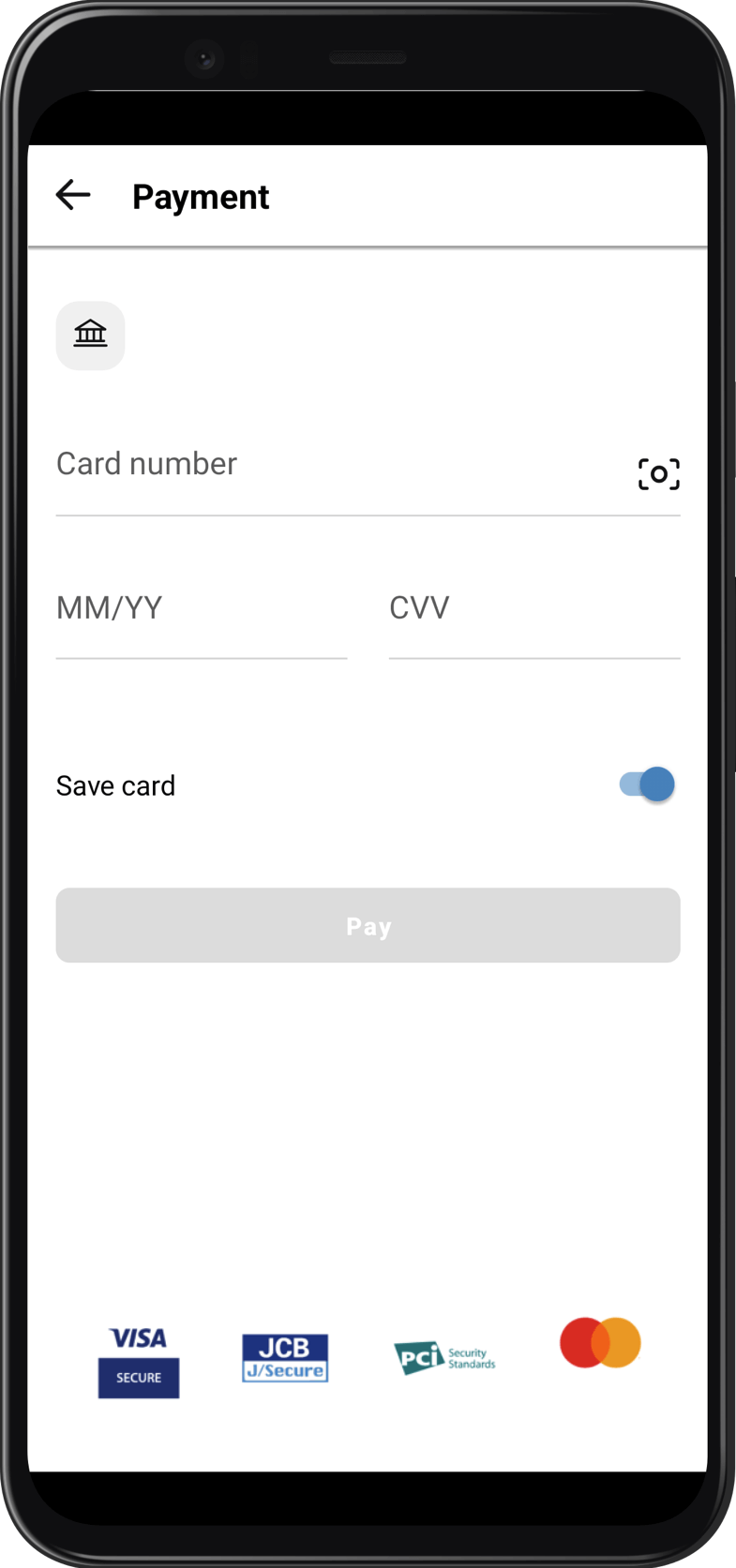
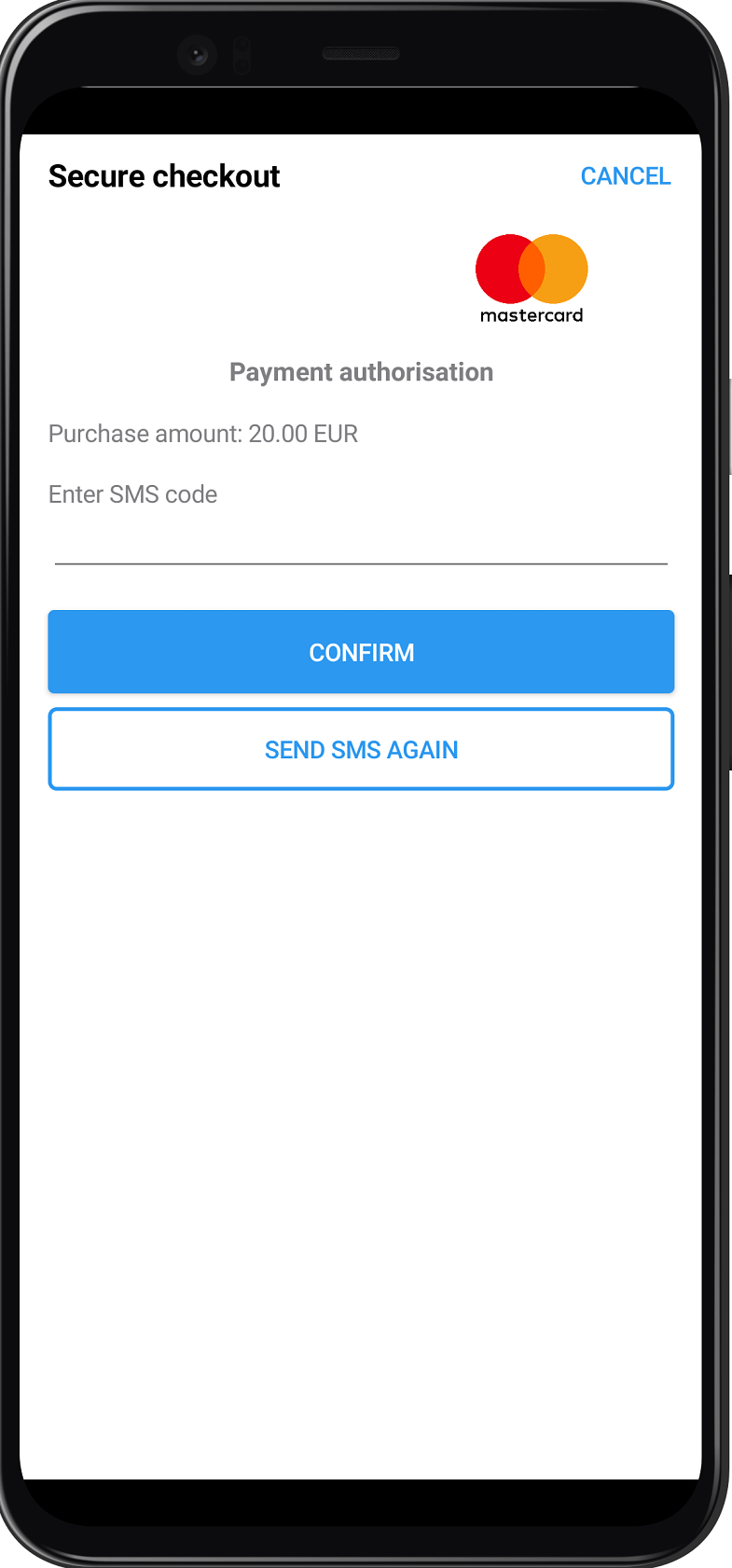
Paying with a bound card
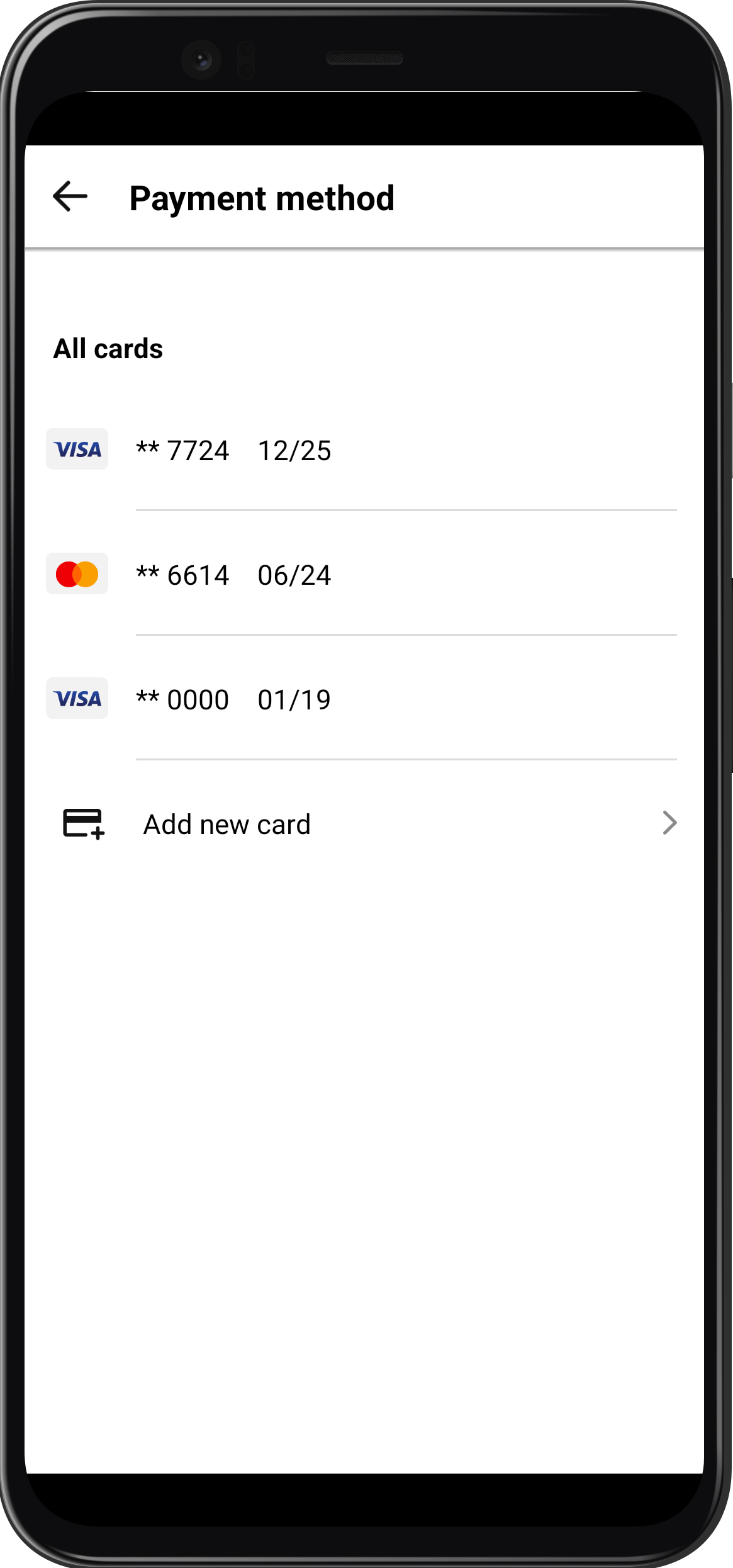
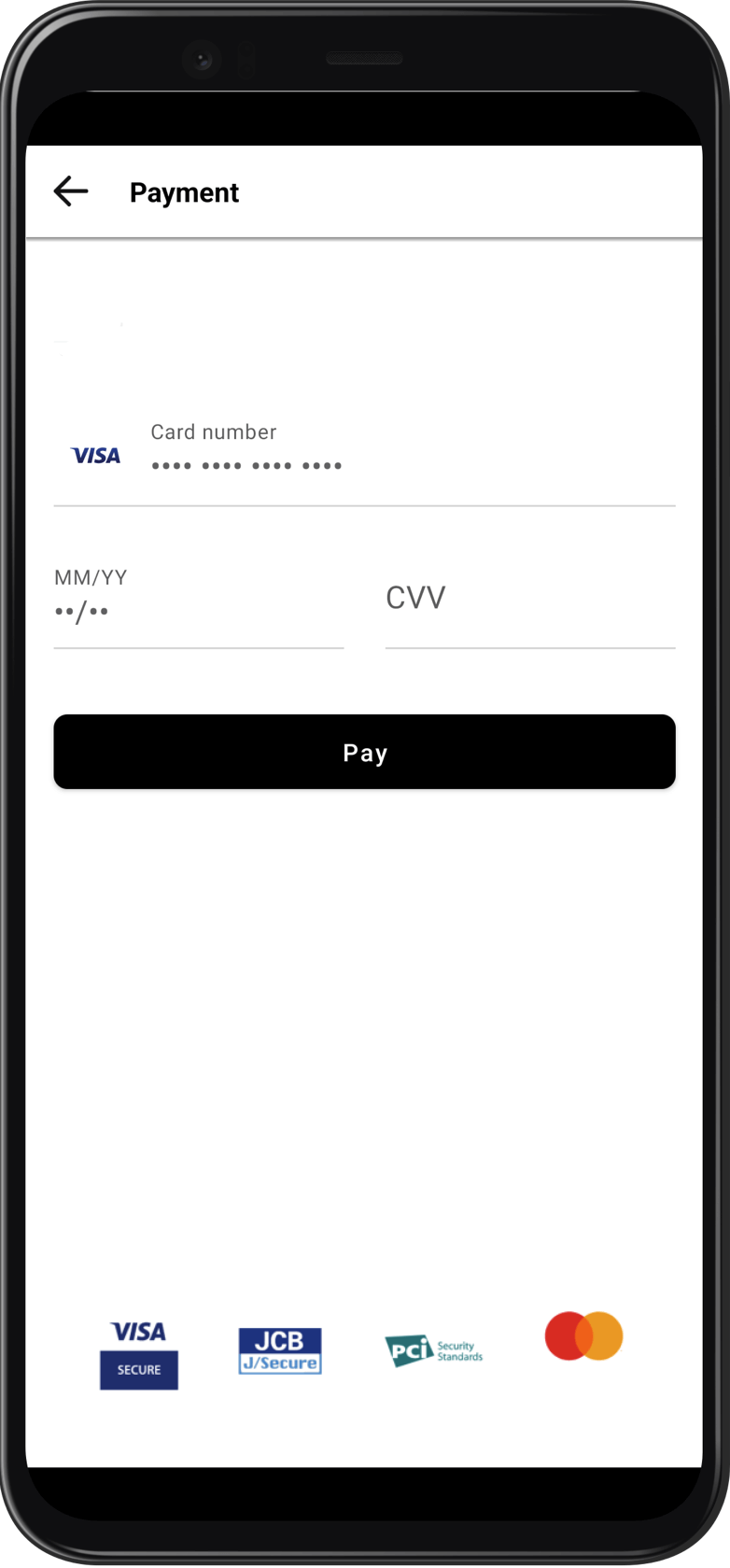
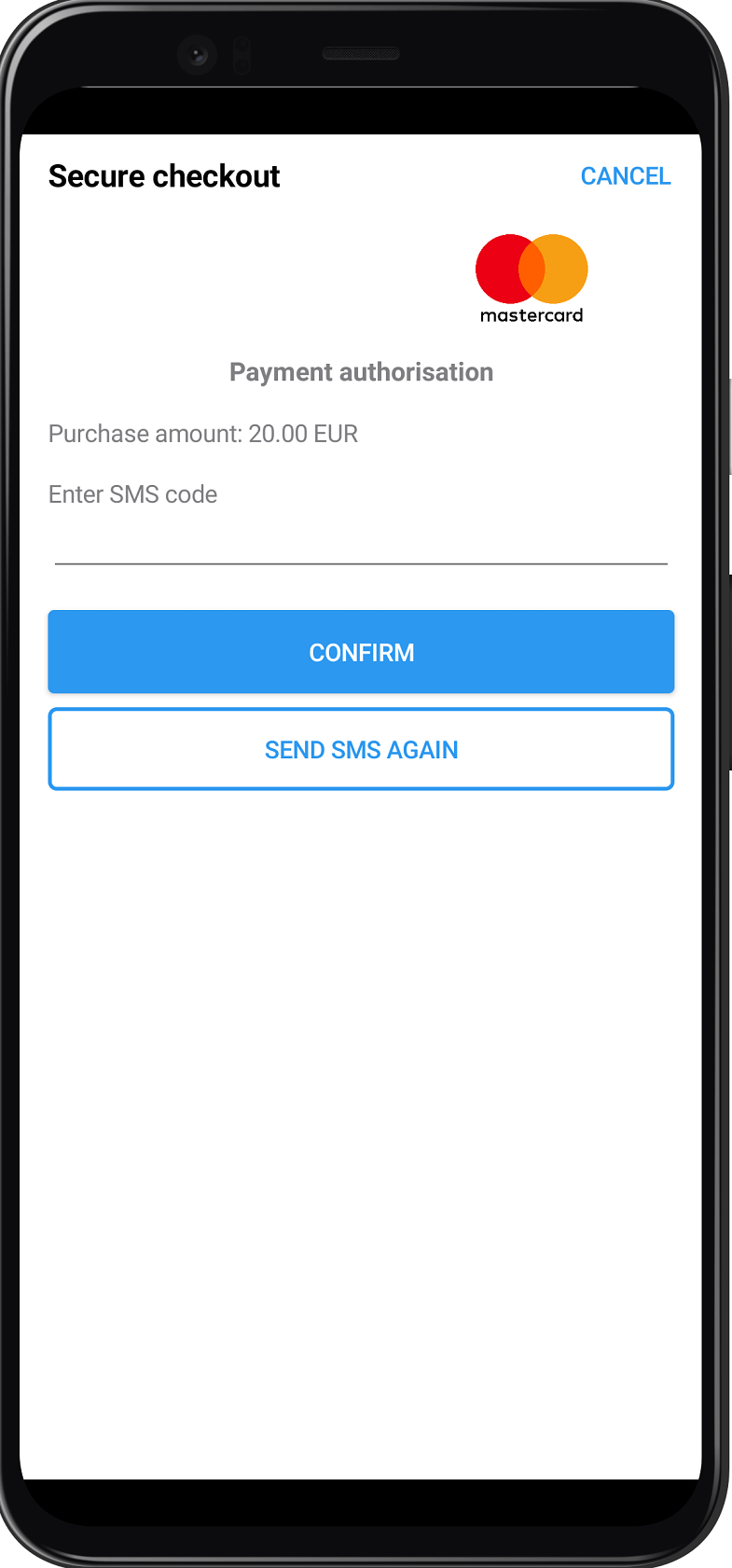
Payment via Google pay by the SDK Payment module
To make a payment via Google Pay by the SDK Payment module, you need to call the
checkout()
payment method while passing true
value of the gPayClicked
flag. The default value is false
.
fun checkout(activity: Activity, checkoutConfig: CheckoutConfig, gPayClicked: Boolean = false) {
}
Wallet button screens
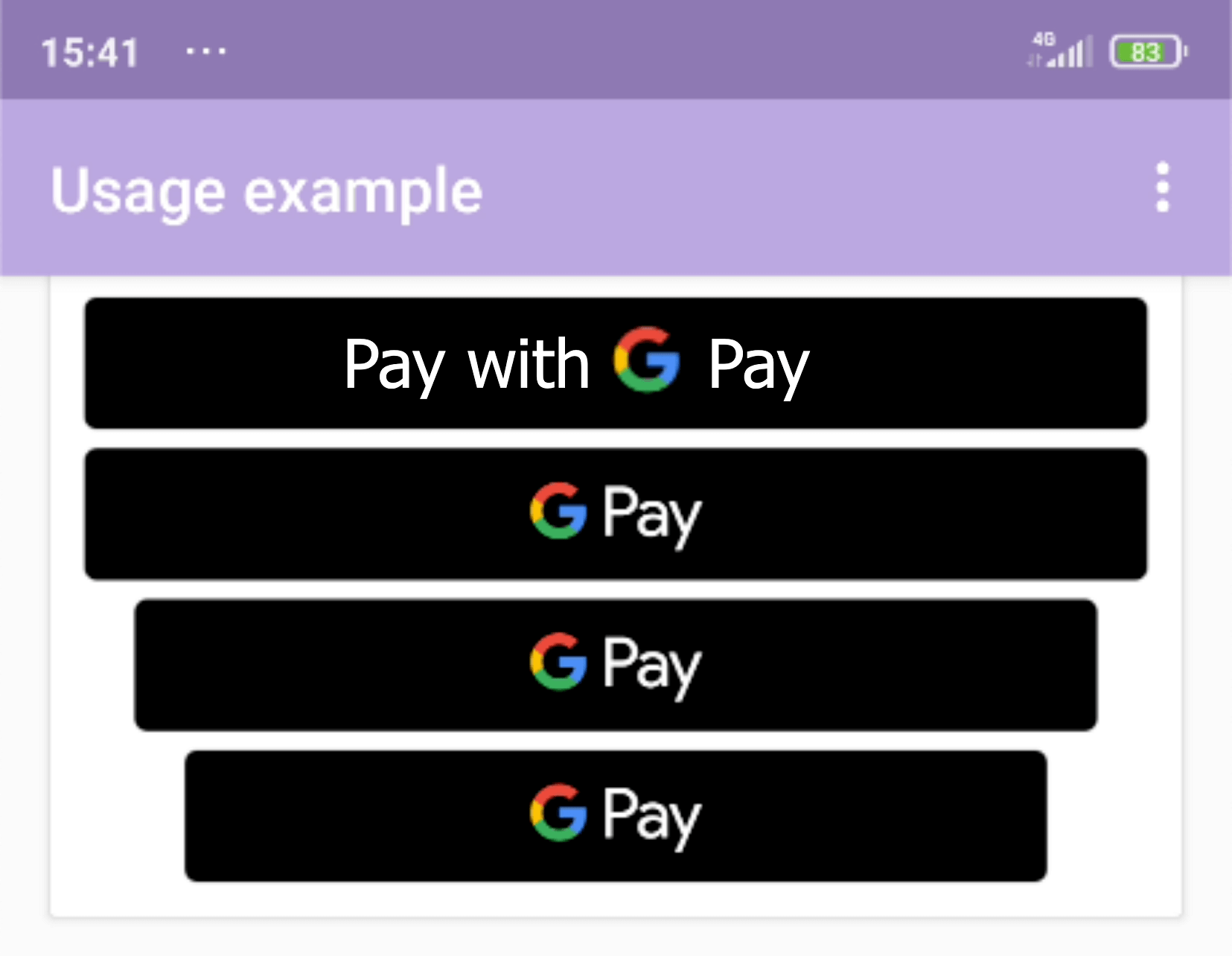
Logging
Internal processes are logged with the SDK-Core
tag.
You can also log your processes.
Logging is available through the Logger
object.
-
To add log- interfaces you should call the
Logger
-methodaddLogInterface()
.Example of logging into LogCat:
...
Logger.addLogInterface(object : LogInterface {
override fun log(classMethod: Class<Any>, tag: String, message: String, exception: Exception?) {
Log.i(tag, "$classMethod: $message", exception)
}
})
...
The default tag is SDK-Core
. You can set your own one if you like.
- To log your own events, you should call
Logger
-methodlog()
.
Example:
...
Logger.log(this.javaClass, "MyTag", "My process...", null)
...
FAQ
Q: What is CardIOUtilities?
A: CardIOUtilities
is an interface of the CardIO
lib. You can read a manual here https://github.com/card-io/card.io-iOS-source.